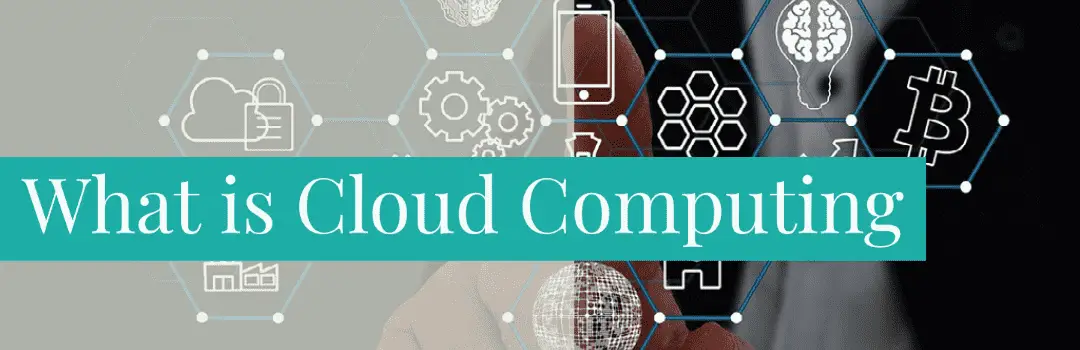
10
Apr
Understanding the URI Parameter and Query Parameter
How many times have you wondered whether to use query parameter or URI parameter when designing an API. Lets understand the difference.
As an API developer, you would have designed an API to get a collection of resource, filter the resource or do some action on an instance resource. It is important to understand when to use a query parameter and uri parameter. They both serve different purpose.
Unique Resource Identifier (URI) is a resource identifier passed as a parameter in the Uniform Resource Locator (URL). Unique Resource Identifier (URI) identifies a unique instance of a particular resource type. The URI parameter should be a unique identifier.
Example
Get an account with unique id 1234
GET /accounts/{accountId}
GET /accounts/1234
Get a book with id 234
GET /books/{bookId}
GET /books/234
JAX-RS example
@Path("/accounts/{account-id}")
public class UserResource {
@GET
@Produces("application/json")
public String getAccount(@PathParam("account-id") String accountId) {
...
}
}
RAML Example
/accounts:
get:
description: retrieve all accounts with a possibility to limit the number of accounts in the response.
is: [paged, filterable]
responses:
200:
body:
type: account[]
example: !include account-example/AccountsExample.raml
/{externalId}:
get:
description: Retrieve an account by specifying the external Id
responses:
200:
body:
type: account
example: !include account-example/AccountExample.raml
404:
body:
example:
message: Not account found for the external id
Query parameters are used to filter the collection resources type. The query parameters are passed at the end of the URL after a question mark to sort, filter or paginate the resource.
Example
Get the accounts which are in active state and limit it by 50 accounts
/accounts?status=active&limit=50
/books?year=2021&limit=20&sort=ASC
In the example above the query parameters are used to sort, filter and paginate the collection of result.
JAX-RS example
@Path("/accounts")
public class UserService {
@GET
@Path("/query")
public Response getAccounts(
@QueryParam("status") string status){
}}
RAML Example
#%RAML 1.0 Trait
queryParameters:
accountName:
displayName: Account type
description: Filter accounts based on account type
type: string
required: false
example: personal
#%RAML 1.0 Trait
queryParameters:
limit:
displayName: Limit
description: Specify the limit of accounts retrieved per page
type: integer
required: false
example: 80
default: 10
offset:
displayName: Offset
description: Specify the page that you want to retrieve
type: integer
required: false
example: 1
default: 0
So what is the best practice?
Best practice is to use URI parameter to identify nad get aunique resource type under a collection. And use query paramter to sort, filter and paginate resources.
TLDR:
Query Parameters: Use query parameters or query string to sort, filter and paginate resources.
URI Parameter: Use URI parameter to get a unique resource type.